Visualization¶
Projection plot¶
Produce a projection image()
plot of density.
>>> import plonk
>>> snap = plonk.load_snap('disc_00030.h5')
>>> snap.image(quantity='density')
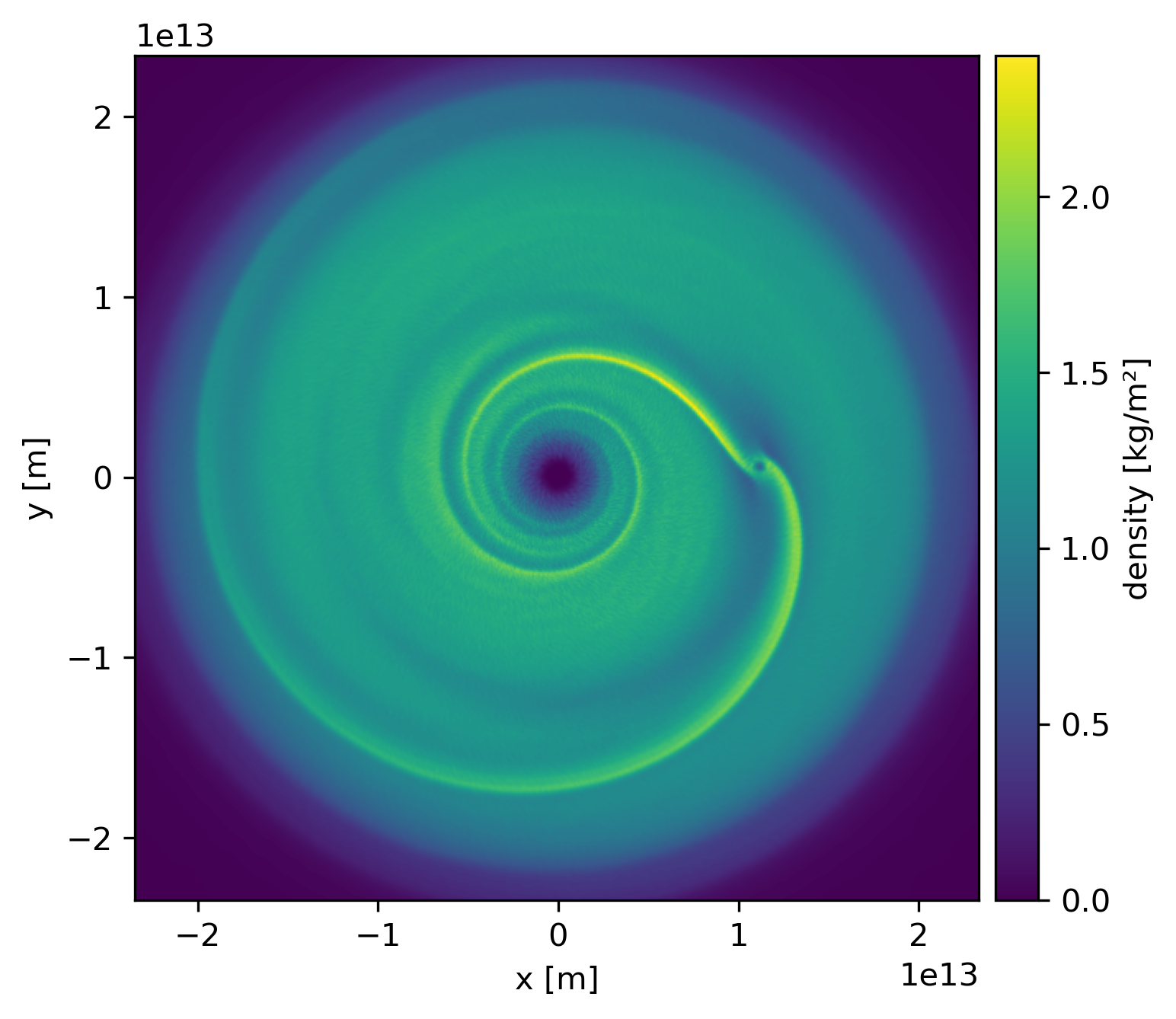
Set plot units, extent, colormap, and colorbar range.
>>> import plonk
>>> snap = plonk.load_snap('disc_00030.h5')
>>> units = {'position': 'au', 'density': 'g/cm^3', 'projection': 'cm'}
>>> snap.image(
... quantity='density',
... extent=(20, 120, -50, 50),
... units=units,
... cmap='gist_heat',
... vmin=0.1,
... vmax=0.2,
... )
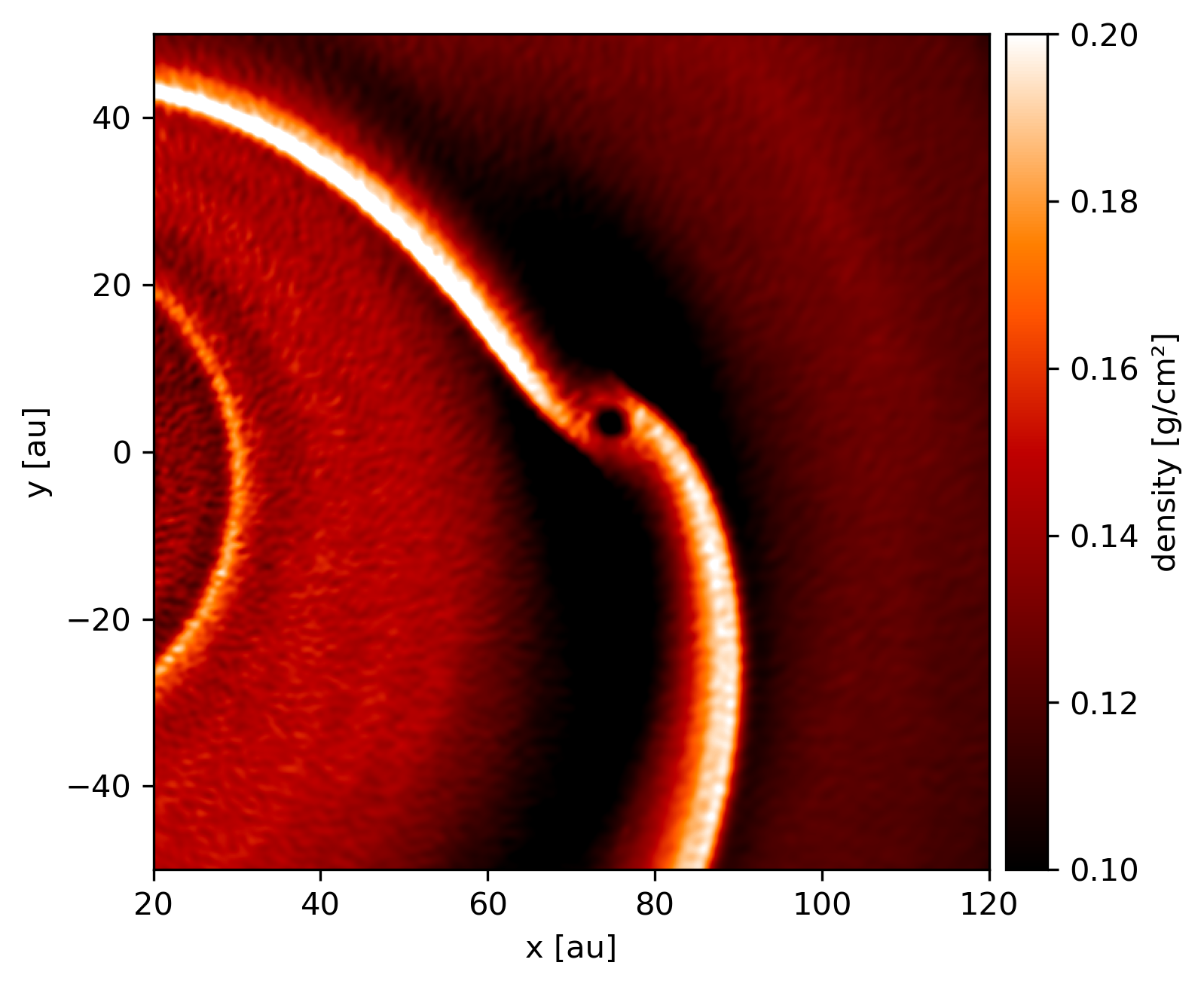
You can set the units on the Snap
instead of passing into the
image()
method.
>>> snap.set_units(position='au', density='g/cm^3', projection='cm')
>>> snap.image(
... quantity='density',
... extent=(20, 120, -50, 50),
... cmap='gist_heat',
... vmin=0.1,
... vmax=0.2,
... )
Cross-section plot¶
Produce a cross-section image()
plot of density.
>>> import plonk
>>> snap = plonk.load_snap('disc_00030.h5')
>>> snap.set_units(position='au', density='g/cm^3')
>>> snap.image(
... quantity='density',
... x='x',
... y='z',
... interp='slice',
... cmap='gist_heat',
... )
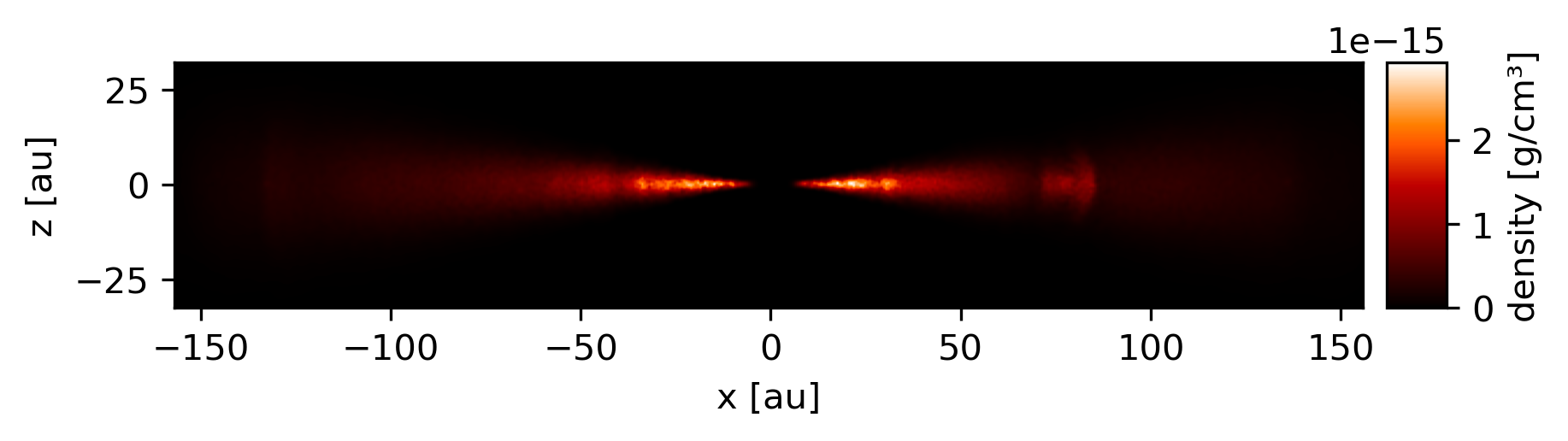
Particle plot¶
Produce a plot of the particles using plot()
with z-coordinate on
the x-axis and smoothing length on the y-axis.
The different colours refer to different particle types.
>>> import plonk
>>> snap = plonk.load_snap('disc_00030.h5')
>>> snap.set_units(position='au', smoothing_length='au')
>>> snap.plot(x='z', y='h', alpha=0.1)
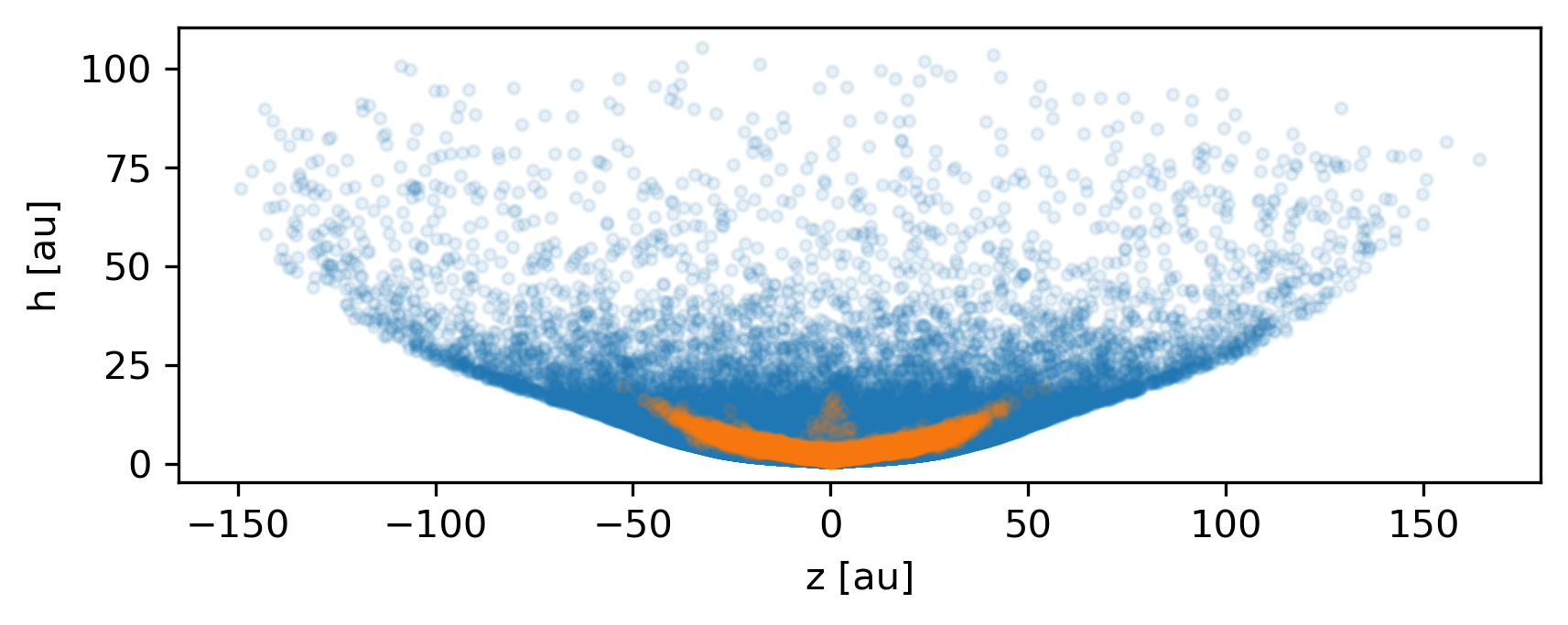
Plot particles with color representing density.
>>> import plonk
>>> snap = plonk.load_snap('disc_00030.h5')
>>> snap.set_units(position='au', density='g/cm^3')
>>> ax = snap.plot(
... x='x',
... y='z',
... c='density',
... xlim=(-50, 50),
... ylim=(-20, 20),
... )
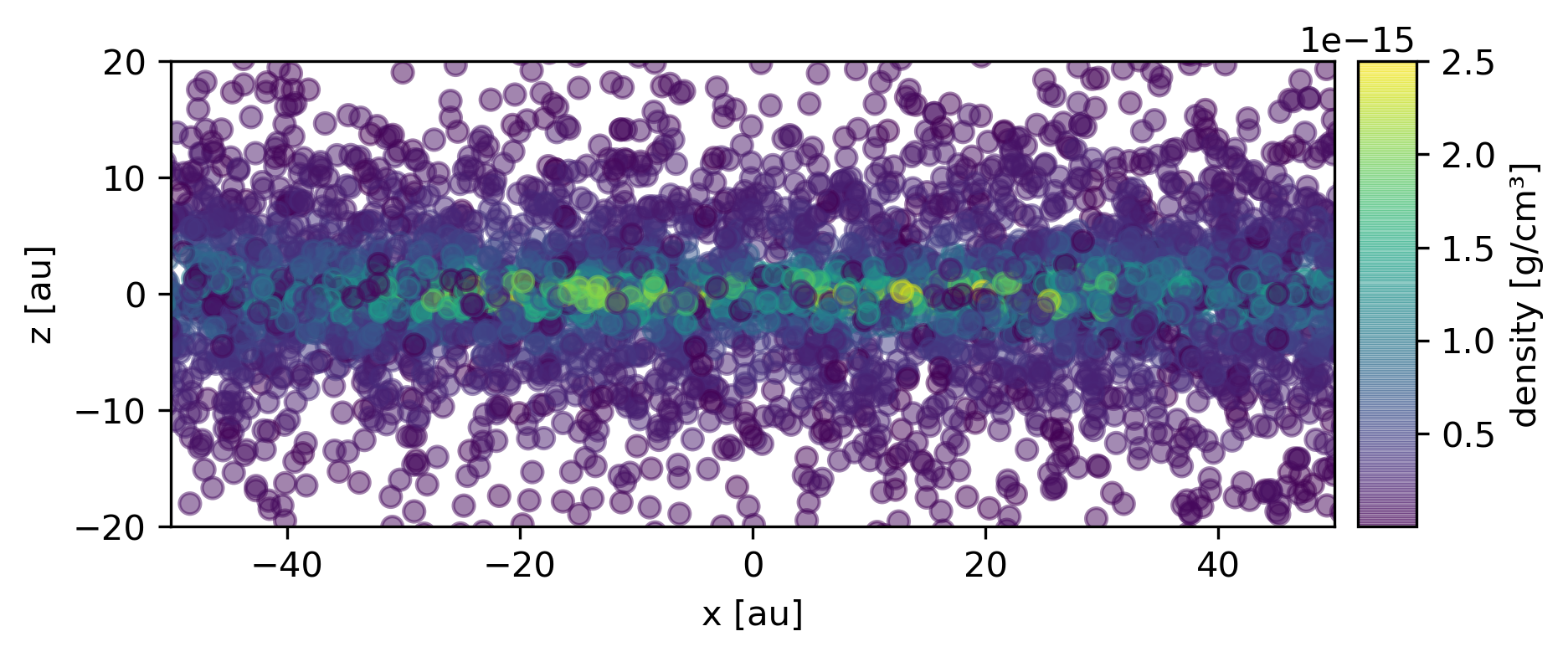
Animations¶
Produce an animation of images using animate()
.
>>> import plonk
>>> sim = plonk.load_simulation(prefix='disc')
>>> units={'position': 'au', 'density': 'g/cm^3', 'projection': 'cm'}
>>> plonk.animate(
... filename='animation.mp4',
... snaps=sim.snaps,
... quantity='density',
... extent=(-160, 160, -160, 160),
... units=units,
... adaptive_colorbar=False,
... save_kwargs={'fps': 10, 'dpi': 300},
... )